Note
Click here to download the full example code
Simple Shaun Example
A simple example showing how to apply Shaun to correct for atmospheric seeing in a region of an SST/CRISP image.
import matplotlib.pyplot as plt
import numpy as np
from crispy import CRISP
from smug.shaun_inference import pretrained_shaun_corrector
Load data, rotate it to fill the frame, and select sub-region to work on.
data = CRISP("crisp_l2_20140906_152724_6563_r00447_3wavelengths.fits")
data.rotate_crop()
crisp_sub_region = data[:, 200:500, 200:500]
/opt/hostedtoolcache/Python/3.9.13/x64/lib/python3.9/site-packages/astropy/wcs/wcs.py:725: FITSFixedWarning: 'datfix' made the change 'Set MJD-AVG to 56906.703926 from DATE-AVG'.
warnings.warn(
/opt/hostedtoolcache/Python/3.9.13/x64/lib/python3.9/site-packages/astropy/io/fits/card.py:264: VerifyWarning: Keyword name 'frame_dims' is greater than 8 characters or contains characters not allowed by the FITS standard; a HIERARCH card will be created.
warnings.warn(
/opt/hostedtoolcache/Python/3.9.13/x64/lib/python3.9/site-packages/astropy/wcs/wcs.py:725: FITSFixedWarning: 'datfix' made the change 'Set MJD-AVG to 56906.703926 from DATE-AVG'.
warnings.warn(
Construct Shaun model and apply to image.
corrector = pretrained_shaun_corrector("Halpha")
shaun_im = corrector.correct_image(crisp_sub_region.data)
Downloading: "https://www.astro.gla.ac.uk/users/USER-MANAGED/solar_model_weights/Shaun_Halpha_1.0.0.pth.tar" to /home/runner/.cache/torch/hub/checkpoints/Shaun_Halpha_1.0.0.pth.tar
0%| | 0.00/131M [00:00<?, ?B/s]
0%| | 32.0k/131M [00:00<08:43, 262kB/s]
0%| | 64.0k/131M [00:00<08:25, 271kB/s]
0%| | 96.0k/131M [00:00<08:18, 275kB/s]
0%| | 128k/131M [00:00<08:02, 284kB/s]
0%| | 192k/131M [00:00<05:57, 383kB/s]
0%| | 272k/131M [00:00<04:39, 489kB/s]
0%| | 368k/131M [00:00<03:46, 602kB/s]
0%| | 480k/131M [00:00<03:09, 720kB/s]
0%| | 608k/131M [00:01<02:41, 843kB/s]
1%| | 768k/131M [00:01<02:14, 1.01MB/s]
1%| | 976k/131M [00:01<01:48, 1.25MB/s]
1%| | 1.19M/131M [00:01<01:30, 1.50MB/s]
1%|1 | 1.45M/131M [00:01<01:17, 1.76MB/s]
1%|1 | 1.78M/131M [00:01<01:04, 2.10MB/s]
2%|1 | 2.16M/131M [00:01<00:54, 2.48MB/s]
2%|2 | 2.62M/131M [00:01<00:45, 2.98MB/s]
2%|2 | 3.20M/131M [00:02<00:36, 3.63MB/s]
3%|3 | 3.98M/131M [00:02<00:28, 4.63MB/s]
4%|3 | 5.02M/131M [00:02<00:21, 5.99MB/s]
5%|4 | 6.41M/131M [00:02<00:16, 7.91MB/s]
6%|6 | 8.09M/131M [00:02<00:12, 10.0MB/s]
8%|7 | 9.88M/131M [00:02<00:10, 11.8MB/s]
9%|8 | 11.6M/131M [00:02<00:09, 13.0MB/s]
10%|# | 13.4M/131M [00:02<00:08, 13.8MB/s]
12%|#1 | 15.2M/131M [00:02<00:08, 14.4MB/s]
13%|#2 | 17.0M/131M [00:03<00:08, 14.8MB/s]
14%|#4 | 18.7M/131M [00:03<00:07, 15.1MB/s]
16%|#5 | 20.5M/131M [00:03<00:07, 15.0MB/s]
17%|#7 | 22.2M/131M [00:03<00:07, 15.2MB/s]
18%|#8 | 24.0M/131M [00:03<00:07, 15.3MB/s]
20%|#9 | 25.8M/131M [00:03<00:07, 15.5MB/s]
21%|##1 | 27.5M/131M [00:03<00:06, 15.5MB/s]
22%|##2 | 29.3M/131M [00:03<00:06, 15.5MB/s]
24%|##3 | 31.0M/131M [00:04<00:06, 15.4MB/s]
25%|##5 | 32.8M/131M [00:04<00:06, 15.5MB/s]
26%|##6 | 34.5M/131M [00:04<00:06, 15.4MB/s]
28%|##7 | 36.3M/131M [00:04<00:06, 15.6MB/s]
29%|##9 | 38.0M/131M [00:04<00:06, 15.6MB/s]
30%|### | 39.8M/131M [00:04<00:06, 15.6MB/s]
32%|###1 | 41.5M/131M [00:04<00:06, 15.5MB/s]
33%|###3 | 43.3M/131M [00:04<00:05, 15.6MB/s]
34%|###4 | 45.1M/131M [00:04<00:05, 15.6MB/s]
36%|###5 | 46.8M/131M [00:05<00:05, 15.5MB/s]
37%|###7 | 48.5M/131M [00:05<00:05, 15.3MB/s]
38%|###8 | 50.2M/131M [00:05<00:05, 15.2MB/s]
40%|###9 | 51.9M/131M [00:05<00:05, 15.3MB/s]
41%|####1 | 53.7M/131M [00:05<00:05, 15.4MB/s]
42%|####2 | 55.4M/131M [00:05<00:05, 15.4MB/s]
44%|####3 | 57.1M/131M [00:05<00:05, 15.4MB/s]
45%|####5 | 58.9M/131M [00:05<00:04, 15.5MB/s]
46%|####6 | 60.6M/131M [00:06<00:04, 15.4MB/s]
48%|####7 | 62.4M/131M [00:06<00:04, 15.5MB/s]
49%|####9 | 64.1M/131M [00:06<00:04, 15.6MB/s]
50%|##### | 65.9M/131M [00:06<00:04, 15.5MB/s]
52%|#####1 | 67.6M/131M [00:06<00:04, 15.5MB/s]
53%|#####3 | 69.4M/131M [00:06<00:04, 15.5MB/s]
54%|#####4 | 71.1M/131M [00:06<00:04, 15.5MB/s]
56%|#####5 | 72.8M/131M [00:06<00:03, 15.5MB/s]
57%|#####7 | 74.6M/131M [00:06<00:03, 15.5MB/s]
58%|#####8 | 76.3M/131M [00:07<00:03, 15.5MB/s]
60%|#####9 | 78.0M/131M [00:07<00:03, 15.4MB/s]
61%|######1 | 79.8M/131M [00:07<00:03, 15.5MB/s]
62%|######2 | 81.5M/131M [00:07<00:03, 15.5MB/s]
64%|######3 | 83.3M/131M [00:07<00:03, 14.7MB/s]
65%|######5 | 85.1M/131M [00:07<00:03, 15.0MB/s]
66%|######6 | 86.9M/131M [00:07<00:03, 15.2MB/s]
68%|######7 | 88.6M/131M [00:07<00:02, 15.3MB/s]
69%|######9 | 90.4M/131M [00:08<00:02, 15.4MB/s]
70%|####### | 92.1M/131M [00:08<00:02, 15.4MB/s]
72%|#######1 | 93.9M/131M [00:08<00:02, 15.5MB/s]
73%|#######3 | 95.6M/131M [00:08<00:02, 15.6MB/s]
75%|#######4 | 97.4M/131M [00:08<00:02, 15.6MB/s]
76%|#######5 | 99.1M/131M [00:08<00:02, 15.6MB/s]
77%|#######7 | 101M/131M [00:08<00:01, 15.6MB/s]
79%|#######8 | 103M/131M [00:08<00:01, 15.6MB/s]
80%|#######9 | 104M/131M [00:08<00:01, 15.6MB/s]
81%|########1 | 106M/131M [00:09<00:01, 15.6MB/s]
83%|########2 | 108M/131M [00:09<00:01, 15.6MB/s]
84%|########3 | 110M/131M [00:09<00:01, 15.6MB/s]
85%|########5 | 111M/131M [00:09<00:01, 15.6MB/s]
87%|########6 | 113M/131M [00:09<00:01, 15.7MB/s]
88%|########7 | 115M/131M [00:09<00:01, 15.6MB/s]
89%|########9 | 117M/131M [00:09<00:00, 15.5MB/s]
91%|######### | 118M/131M [00:09<00:00, 15.6MB/s]
92%|#########1| 120M/131M [00:10<00:00, 15.6MB/s]
93%|#########3| 122M/131M [00:10<00:00, 15.6MB/s]
95%|#########4| 124M/131M [00:10<00:00, 15.6MB/s]
96%|#########5| 125M/131M [00:10<00:00, 15.6MB/s]
97%|#########7| 127M/131M [00:10<00:00, 15.6MB/s]
99%|#########8| 129M/131M [00:10<00:00, 15.5MB/s]
100%|#########9| 131M/131M [00:10<00:00, 15.4MB/s]
100%|##########| 131M/131M [00:10<00:00, 12.7MB/s]
loading Shaun model
=> model loaded.
Segmenting image cube: 0%| | 0/3 [00:00<?, ?it/s]
Segmenting image cube: 100%|##########| 3/3 [00:00<00:00, 1402.31it/s]
Compare line-core corrected image to one with original atmospheric seeing.
fig, ax = plt.subplots(1, 2)
ax[0].imshow(crisp_sub_region[1].data, cmap="Greys_r")
ax[0].set_title("Original Data")
ax[1].imshow(shaun_im[1], cmap="Greys_r")
ax[1].set_title("Shaun Corrected")
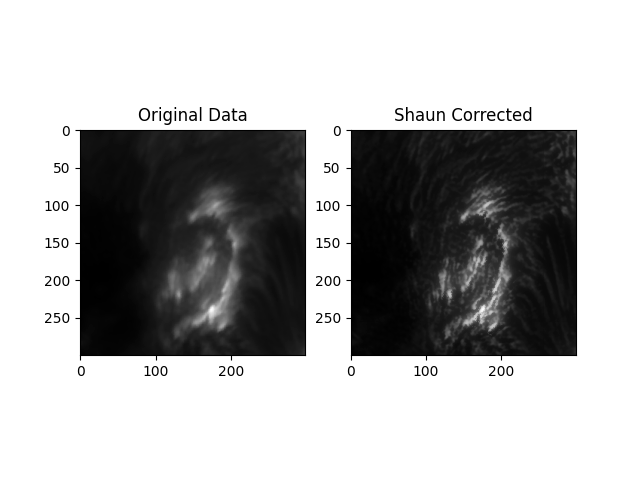
Text(0.5, 1.0, 'Shaun Corrected')
Total running time of the script: ( 0 minutes 30.225 seconds)